Project Tutorials
Introduction Directory Structure Project Setup Remove index.php using htaccess Change Default Controller CSS Add in Project Create Login Page Design Validation on Login Login with Database Flashdata on Login Display Username after Login Logout Design Dashboard Page List user data form database Insert Data Flash message show if data inserted Delete data from datebase Edit data from datebase Active Inactive user status Show active users detail on home page Image Upload Edit Image View Image on Home PageList user data form database
In this tutorials, we are going to learn how to fetch user details from database.
Step 1 : Create table in database.
CREATE TABLE `ci_project`.`userdata` ( `id` INT NOT NULL AUTO_INCREMENT , `username` VARCHAR(300) NOT NULL , `email` VARCHAR(300) NOT NULL , `contact` VARCHAR(100) NOT NULL , `status` INT(11) NOT NULL , PRIMARY KEY (`id`)) ENGINE = InnoDB;
Step 2 : Create dashboard model in model folder and then we have to write the query in it so that we can fetch the data from the table of users.
<?php
class Dashboard_model extends CI_Model
{
public function user_details()
{
$query = $this->db->select('id,username,email,contact,status')
->from('userdata')
->order_by('id','desc')
->get();
return $query->result();
}
}
?>
Step 3 : Now we will fetch the data from the model in the controller and pass it to view page where we can show the details of the user.
so firstly, load the dashboard model in controller and we will give him the useradata name.
eg : $this->load->model('dashboard_model','userdata');
public function index()
{
$this->load->model('dashboard_model','userdata');
$user_list = $this->userdata->user_details();
$this->load->view('dashboard',['userdata'=>$user_list]);
}
Step 4 : Now we will use foreach loop. So that we can show the details of the user on the view page.
foreach : In foreach loop works only on arrays and objects.
eg:
<table class="table table-striped" style="margin-top: 20px;">
<thead>
<tr>
<th>S.no</th>
<th>Name</th>
<th>Email</th>
<th>Contact</th>
<th>Status</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<?php
$i = 1;
foreach ($userdata as $users) {
?>
<tr>
<td><?php echo $i; ?></td>
<td><?php echo $users->username; ?></td>
<td><?php echo $users->email; ?></td>
<td><?php echo $users->contact; ?></td>
<td>
<button class="btn btn-success">Active</button>
</td>
<td>
<button class="btn btn-warning">Edit</button>
<button class="btn btn-danger">Delete</button>
</td>
</tr>
<?php $i++; } ?>
</tbody>
</table>
Result :
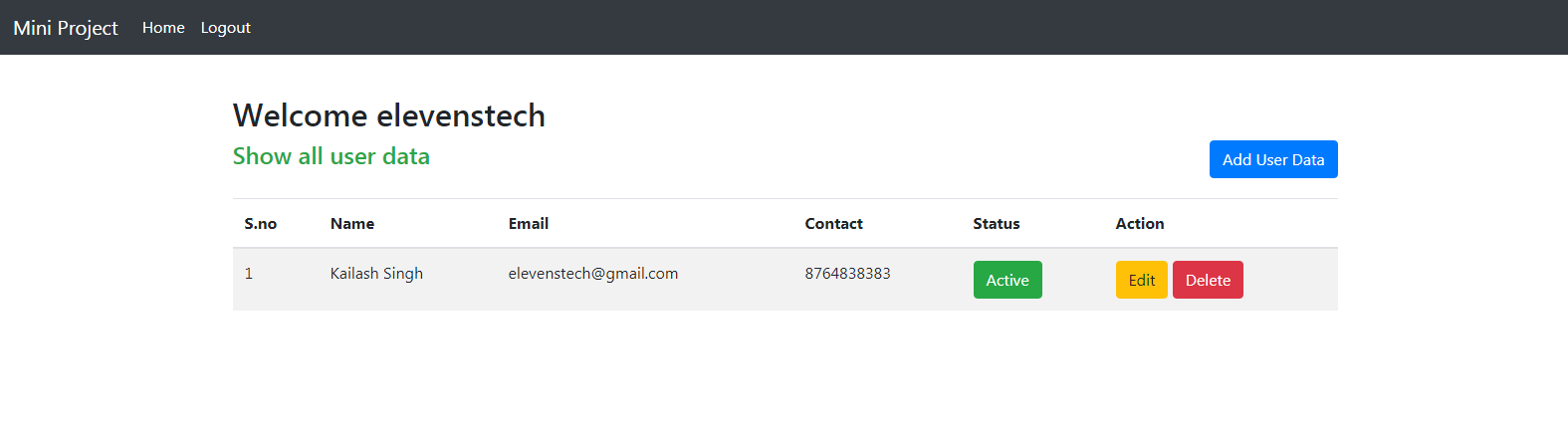
Source Code:
Codeigniter tutorial for beginners
In this project. We are providing you, how to create small project in Codeign....
Source CodeElevenstech Web Tutorials
Elevenstech Web Tutorials helps you learn coding skills and enhance your skills you want.
As part of Elevenstech's Blog, Elevenstech Web Tutorials contributes to our mission of “helping people learn coding online”.
Read More
Newsletter
Subscribe to get the latest updates from Elevenstech Web Tutorials and stay up to date