Project Tutorials
Introduction Directory Structure Project Setup Remove index.php using htaccess Change Default Controller CSS Add in Project Create Login Page Design Validation on Login Login with Database Flashdata on Login Display Username after Login Logout Design Dashboard Page List user data form database Insert Data Flash message show if data inserted Delete data from datebase Edit data from datebase Active Inactive user status Show active users detail on home page Image Upload Edit Image View Image on Home PageValidation on Login
In this tutorial, we are going to create validation on login page. When you submit login form.
Step 1 : Load helper file in Login Controller.
Helper : It is a simply a collection of functions in a particular category. Each helper function performs one specific task. Codeigniter does not load helper files by default, so the first step in using a helper is to load it.
eg:
<?php
class Login extends CI_Controller {
public function index()
{
// here i am loading helper file, which is perform a specific task
$this->load->helper('form');
$this->load->view('login');
}
}
?>
Now, also change form code in login page in views folder.
<?php echo form_open('login'); ?>
<?php echo form_input(['name'=>'username','class'=>'form-control','placeholder'=> 'Enter Username']); ?>
<?php echo form_input(['name'=>'password','class'=>'form-control','placeholder'=> 'Enter Password']); ?>
<?php echo form_submit(['name'=>'submit','class'=>'btn btn-primary','value'=> 'Login']); ?>
</form>
Step 2 : Create form validation on login form submit. for login validation, we are using form_validation library in Login controller.
eg:
<?php
class Login extends CI_Controller {
public function index()
{
$this->load->helper('form');
// here i am loading library file
$this->load->library('form_validation');
$this->load->view('login');
}
}
?>
.
Now, we are adding a form_validation code in login controller to check form value is blank or not.
<?php
class Login extends CI_Controller {
public function index()
{
$this->load->helper('form');
// here i am loading library file
$this->load->library('form_validation');
//here i am adding a rule. If you are not fill the value then it will show the error.
$this->form_validation->set_rules('uname','Username','required');
$this->form_validation->set_rules('password','Password','required');
//Check validation success or not
if($this->form_validation->run())
{
echo'success';
}
else
{
$this->load->view('login');
}
}
}
?>
Step 3 : Now here we will write the code for the warning message, if the user submits without filling the field the they will see the warning message.
eg:
<?php echo form_open('login') ?>
<?php echo form_input(['name'=>'uname','class'=>'form-control','placeholder'=> 'Enter Username']); ?>
//here i am showing. Please fill your username
<?php echo form_error('uname','<p class="text-danger">','</p>'); ?>
<?php echo form_input(['name'=>'password','class'=>'form-control','placeholder'=> 'Enter Password']); ?>
//here i am showing. Please fill your password
<?php echo form_error('password','<p class="text-danger">','</p>'); ?>
<?php echo form_submit(['name'=>'submit','class'=>'btn btn-primary','value'=> 'Login']); ?>
</form>
Result :
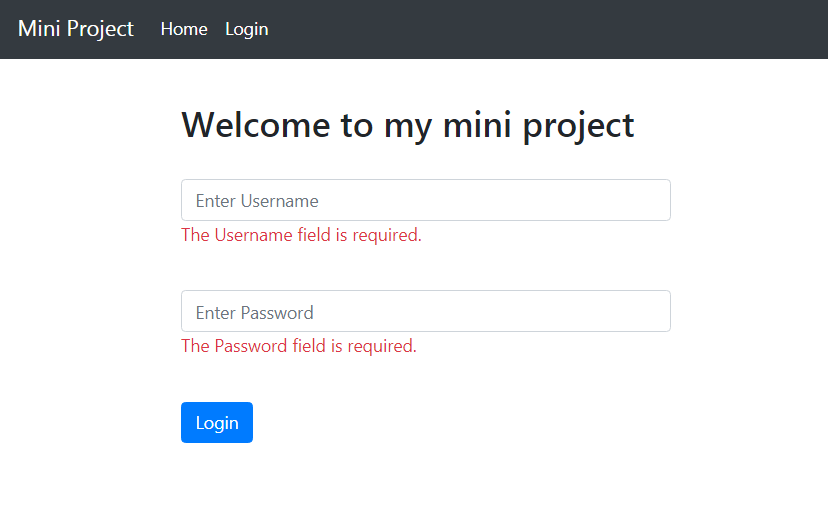
Source Code:
Codeigniter tutorial for beginners
In this project. We are providing you, how to create small project in Codeign....
Source CodeSEARCH POST HERE
Support Us
Subscribe My YouTube Channel
Join Our Telegram Channel & Support Eachother
CATEGORIES
INTERVIEW QUESTIONS
POPULAR POSTS
Elevenstech Web Tutorials
Elevenstech Web Tutorials helps you learn coding skills and enhance your skills you want.
As part of Elevenstech's Blog, Elevenstech Web Tutorials contributes to our mission of “helping people learn coding online”.
Read More
Newsletter
Subscribe to get the latest updates from Elevenstech Web Tutorials and stay up to date