×
×
Image Upload with Progress Bar Using PHP AJAX
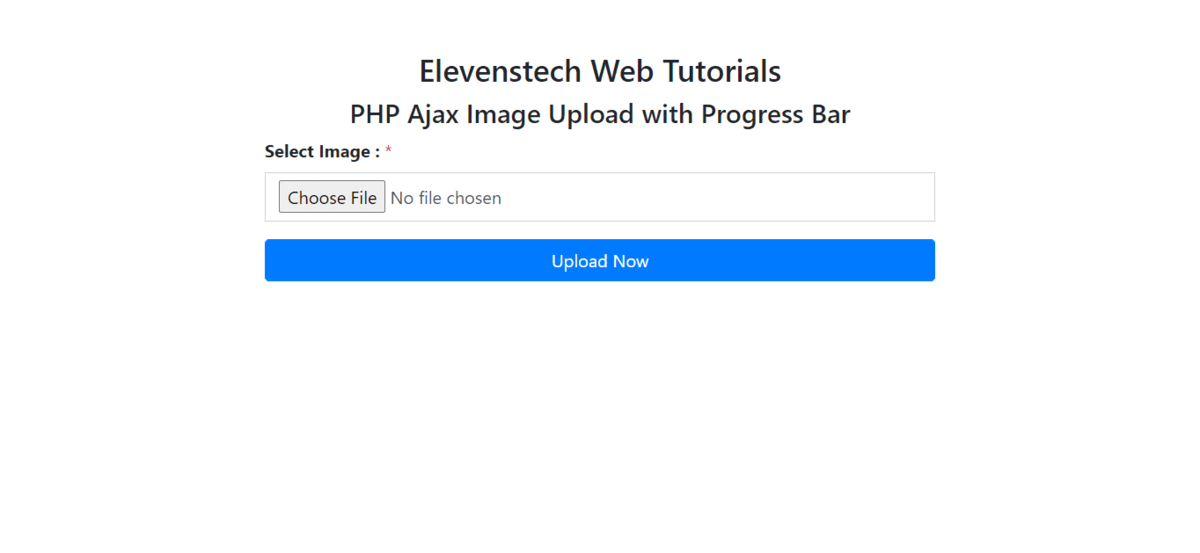
Image Upload with Progress Bar Using PHP AJAX
Published Sep 03,2022 by Kailash Singh
0 Comment 1619 Views
In this tutorial, we are going to teach you, how to create Image Upload with Progress Bar Using PHP AJAX.
This article will give you simple example of Ajax image upload with progress bar with jquery and PHP. We will use get simple upload image and progress bar Jquery AJAX PHP.
Step 1: Create index.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>PHP Ajax Image Upload with Progress Bar - Elevenstech Web Tutorials</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" integrity="sha384-zCbKRCUGaJDkqS1kPbPd7TveP5iyJE0EjAuZQTgFLD2ylzuqKfdKlfG/eSrtxUkn" crossorigin="anonymous">
<script src="https://code.jquery.com/jquery-3.3.1.min.js" type="text/javascript"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery.form/4.3.0/jquery.form.min.js"></script>
<style type="text/css">
.progress {
display: none;
position: relative;
height: 25px;
background-color: #ddd;
border-radius: 15px;
}
.progress-bar {
background-color: blue;
width: 0%;
height: 30px;
border-radius: 4px;
}
.percent {
position: absolute;
display: inline-block;
color: #fff;
font-weight: bold;
top: 87%;
left: 50%;
margin-top: -9px;
margin-left: -20px;
}
#outputImage {
display: none;
margin-top: 10px;
}
#outputImage img {
max-width: 300px;
}
.form-control{
border-radius: 0px;
height: 45px;
}
</style>
</head>
<body>
<div class="container mt-5">
<div class="row">
<div class="col-md-2"></div>
<div class="col-md-8">
<h3 class="text-center">Elevenstech Web Tutorials</h3>
<h4 class="text-center">PHP Ajax Image Upload with Progress Bar</h4>
<div id='outputImage'></div>
<div class='progress' id="progressDivId">
<div class='progress-bar' id='progressBar'></div>
<div class='percent' id='percent'>0%</div>
</div>
<div id='error'></div>
<form action="upload.php" id="uploadForm" name="frmupload"method="post" enctype="multipart/form-data">
<div class="form-group">
<label><strong>Select Image : </strong><span class="text-danger"> *</span></label>
<input type="file" id="uploadImage" name="uploadImage" class="form-control">
</div>
<div class="d-flex justify-content-center">
<input id="submitButton" type="submit" name='btnSubmit' value="Upload Now" class="btn btn-primary btn-block">
</div>
</form>
</div>
</div>
</div>
<script type="text/javascript">
$(document).ready(function () {
$('#submitButton').click(function () {
$('#uploadForm').ajaxForm({
target: '#outputImage',
url: 'upload.php',
beforeSubmit: function () {
$("#outputImage").hide();
if($("#uploadImage").val() == "") {
$("#outputImage").show();
$("#outputImage").html("<div class='alert alert-danger'>Please select a file</div>");
return false;
}
$("#progressDivId").css("display", "block");
var percentValue = '0%';
$('#progressBar').width(percentValue);
$('#percent').html(percentValue);
},
uploadProgress: function (event, position, total, percentComplete) {
var percentValue = percentComplete + '%';
$("#progressBar").animate({
width: '' + percentValue + ''
}, {
duration: 3000,
easing: "linear",
step: function (x) {
percentText = Math.round(x * 100 / percentComplete);
$("#percent").text(percentText + "%");
if(percentText == "100") {
$("#outputImage").show();
$("#error").html("<div class='alert alert-success mt-2'>File upload successfully.</div>");
}
}
});
},
error: function (response, status, e) {
alert('Oops something went.');
},
complete: function (xhr) {
if (xhr.responseText && xhr.responseText != "error")
{
$("#outputImage").html(xhr.responseText);
}else{
$("#outputImage").show();
$("#outputImage").html("<div class='alert alert-danger'>File uploading problem detected. Please check</div>");
$("#progressBar").stop();
}
}
});
});
});
</script>
</body>
</html>
Step 2: Create upload.php
<?php
if (isset($_POST['btnSubmit'])) {
$uploadfile = $_FILES["uploadImage"]["tmp_name"];
$folderPath = "uploads/";
if (! is_writable($folderPath) || ! is_dir($folderPath)) {
echo "error";
exit();
}
if (move_uploaded_file($_FILES["uploadImage"]["tmp_name"], $folderPath . $_FILES["uploadImage"]["name"])) {
echo '<img src="' . $folderPath . "" . $_FILES["uploadImage"]["name"] . '">';
exit();
}
}
?>
Step 3: Create uploads folder in project.
Comments ( 0 )
Elevenstech Web Tutorials
Elevenstech Web Tutorials helps you learn coding skills and enhance your skills you want.
As part of Elevenstech's Blog, Elevenstech Web Tutorials contributes to our mission of “helping people learn coding online”.
Read More
Newsletter
Subscribe to get the latest updates from Elevenstech Web Tutorials and stay up to date
Copyright 2018 - 2024 Elevenstech Web Tutorials All rights reserved.