Project Tutorials
Introduction of Laravel Setup and Installation of Laravel Project Laravel Structure Create Login Page Design Validation on Login Login with Database Display Username after Login Logout Register Design Dashboard Page Add Product List Products from Database Edit product from database Active Inactive user statusCreate Login Page Design
In this tutorial, we are going to design login page.
Step 1 : Run this link http://localhost/phpmyadmin on browser.
Step 2 : Click on databases and enter database name laravel_project and then click to create.
Step 3 : Go to .env file of laravel project and connect to database.
Now connect the database.
DB_DATABASE=laravel_project
DB_USERNAME=root
DB_PASSWORD=
Step 4 : Create login tables using command.
Open you cmd in project location and enter the command to migrate the tables in database.
php artisan migrate
eg:
Result :
Step 5 : Create seeder to enter the dummy user data in users table.
Now go to your DatabaseSeeder.php file and enter their data of user.
Path : laravel_project/database/seed/DatabaseSeeder.php
Code :
<?php
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
public function run()
{
//enter the user data
DB::table('users')->insert([
'name' => 'Kailash Singh',
'email' => '[email protected]',
'password' => Hash::make('1234567'),
'remember_token' => str_random(10)
]);
}
}
?>
And now run the seeder file on the cmd with the help of this command.
php artisan db:seed
eg:
Step 6 : Create controller for login with the help of command.
php artisan make:controller MainController
eg:
Now your controller has been created,
Step 7 : Load your login page in main controller.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use validator;
use Auth;
class MainController extends Controller
{
function index()
{
//Here i am loading my login page
return view('login');
}
}
?>
Step 8 : Go to your views folder and create login page (login.blade.php).
Path : laravel_project/resources/views/login.blade.php
and then design your login page
<!DOCTYPE html>
<html lang="en">
<head>
<title>Login</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container" style="margin-top: 20px;">
<div class="row">
<div class="col-sm-4"></div>
<div class="col-sm-4">
<h2 style="text-align: center;">Elevenstech Login</h2>
<form method="post" action="#">
<div class="form-group">
<label>Email : </label>
<input type="email" name="email" class="form-control">
</div>
<div class="form-group">
<label>Password : </label>
<input type="password" name="password" class="form-control">
</div>
<button type="submit" name="login" class="btn btn-primary">Login</button>
<p>Don't have an account <a href="#">Register Here</a></p>
</form>
</div>
<div class="col-sm-4"></div>
</div>
</div>
</body>
</html>
Step 9 : Open your web.php file in route folder & change their default view file to login view file
Path : laravel_project/route/web.php
Route::get('/', function () {
return view('login');
});
Step 7 : Now use the command to run your laravel project.
eg : php artisan serve
Now, use this link to run your laravel project on browser
eg : http://localhost:8000/
Click here to download complete code of login
Result :
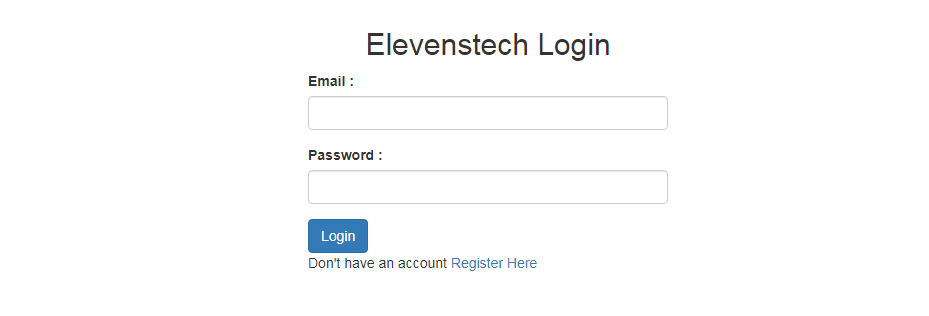
Source Code:
Small Laravel Project
In this project. We are providing you, how to create small project in Laravel....
Source CodeElevenstech Web Tutorials
Elevenstech Web Tutorials helps you learn coding skills and enhance your skills you want.
As part of Elevenstech's Blog, Elevenstech Web Tutorials contributes to our mission of “helping people learn coding online”.
Read More
Newsletter
Subscribe to get the latest updates from Elevenstech Web Tutorials and stay up to date