Project Tutorials
Introduction of Laravel Setup and Installation of Laravel Project Laravel Structure Create Login Page Design Validation on Login Login with Database Display Username after Login Logout Register Design Dashboard Page Add Product List Products from Database Edit product from database Active Inactive user statusList Products from Database
In this tutorials, we are going to teach you how to fetch products from database.
Step 1 : Create successlogin() in main controller and then we have to write the query in it so that we can fetch the data from the table of product.
function successlogin()
{
//Get user id from session
$user_id = Auth::user()->id;
//get product list with the of user id
$products = DB::select('select * from product where user_id = "'.$user_id.'"');
return view('successlogin',['products'=>$products]);
}
Step 2 : Open your dashboard page (successlogin.blade.php) and then we will use foreach loop. So that we can show the details of the products on the dashboard page.
foreach : In foreach loop works only on arrays and objects.
eg :
<table class="table table-striped table-bordered">
<thead class="btn-primary">
<tr>
<th>S.No</th>
<th>Product Name</th>
<th>Product Price</th>
<th>Product Quantity</th>
<th>Status</th>
<th></th>
</tr>
</thead>
<tbody>
<?php
$i = 1;
foreach ($products as $product) {
?>
<tr>
<td><?php echo $i; ?></td>
<td><?php echo $product->product_name; ?></td>
<td><?php echo $product->product_price; ?></td>
<td><?php echo $product->product_quantity; ?></td>
<td>
<?php if($product->status == '1'){ ?>
<a href="#" class="btn btn-success">Active</a>
<?php }else{ ?>
<a href="#" class="btn btn-danger">Inactive</a>
<?php } ?>
</td>
<td>
<a href="{{url('/edit-product',$product->id)}}" class="btn btn-primary">Edit</a>
</td>
</tr>
<?php $i++; } ?>
</tbody>
</table>
Result :
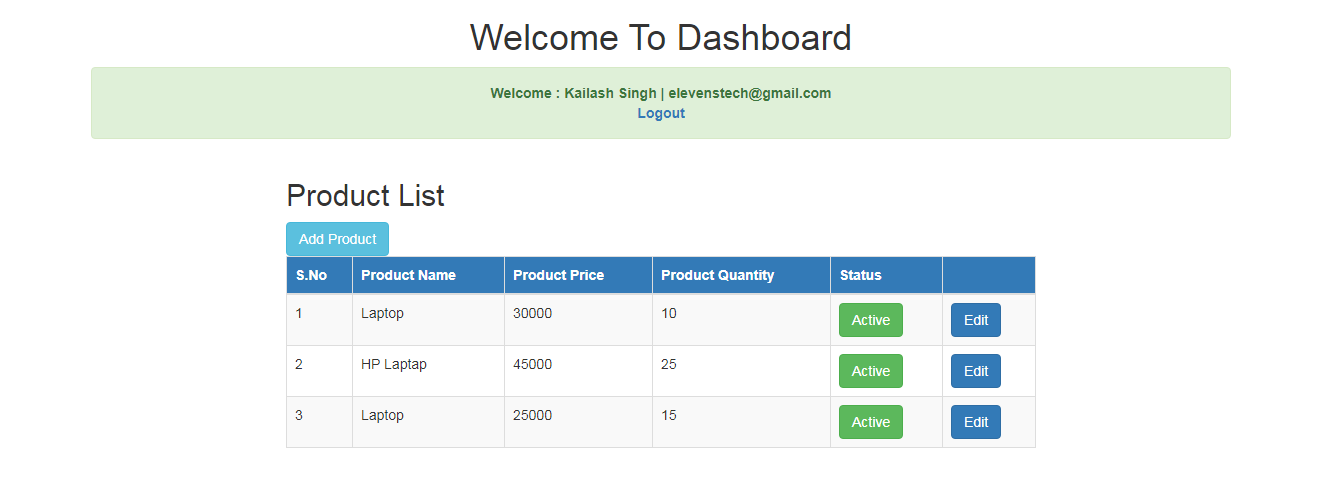
Source Code:
Small Laravel Project
In this project. We are providing you, how to create small project in Laravel....
Source CodeElevenstech Web Tutorials
Elevenstech Web Tutorials helps you learn coding skills and enhance your skills you want.
As part of Elevenstech's Blog, Elevenstech Web Tutorials contributes to our mission of “helping people learn coding online”.
Read More
Newsletter
Subscribe to get the latest updates from Elevenstech Web Tutorials and stay up to date