User Deleted After Confirmation using Codeigniter
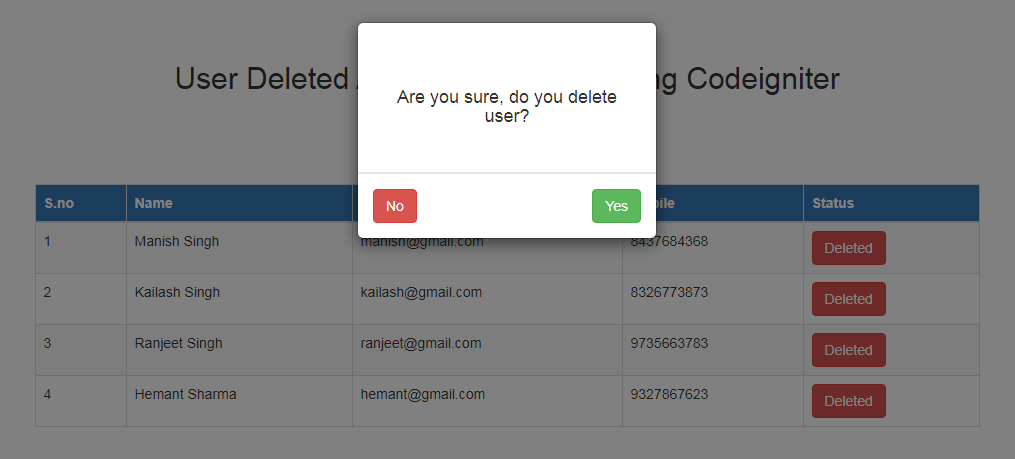
User Deleted After Confirmation using Codeigniter
Published Nov 29,2019 by Kailash Singh
0 Comment 2885 Views
In this tutorial, we are going to learn about how to create User Deleted After Confirmation using Codeigniter.
Step 1:- Create table in database.
CREATE TABLE `codingmantra`.`users` ( `id` INT NOT NULL AUTO_INCREMENT , `name` VARCHAR(250) NOT NULL , `email` VARCHAR(250) NOT NULL , `phone` VARCHAR(100) NOT NULL , `status` ENUM('0','1') NOT NULL , `created_at` TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP , PRIMARY KEY (`id`)) ENGINE = InnoDB;
Step 2:- Insert data in Users table.
INSERT INTO `users` (`id`, `name`, `email`, `phone`, `status`, `created_at`) VALUES (NULL, 'Hemant Sharma', '[email protected]', '9327867623', '1', CURRENT_TIMESTAMP), (NULL, 'Ranjeet Singh', '[email protected]', '9735663783', '1', CURRENT_TIMESTAMP), (NULL, 'Kailash Singh', '[email protected]', '9683683843', '1', CURRENT_TIMESTAMP), (NULL, 'Manish Singh', '[email protected]', '9666466478', '1', CURRENT_TIMESTAMP);
Step 3: Create users controller in controller folder (Users.php)
With the help of this controller, we will pass all the data on the page .
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Users extends CI_Controller {
public function index()
{
$users_list = $this->db->select('*')->from('users')->order_by('id','desc')->get()->result(); //here i'm fetching the data form the table
$this->load->view('users_list',['users_list'=>$users_list]);//load all data in view page
}
}
?>
Step 4: Create users_list page in View folder (users_list.php)
First we will add link on this page
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/js/bootstrap.min.js"></script>
Then list all the users and then we will create a attribute (uid) on the delete button in which we will pass the id of the user.
Example :- <button class="btn btn-danger user_deleted" uid="<?php echo $users->id; ?>">Delete</button>
<table class="table table-bordered table-striped">
<thead>
<tr class="btn-primary">
<th>S.no</th>
<th>Name</th>
<th>Email</th>
<th>Mobile</th>
<th>Status</th>
</tr>
</thead>
<tbody>
<?php
$i = 1;
foreach ($users_list as $users) {
?>
<tr>
<td><?php echo $i; ?></td>
<td><?php echo $users->name; ?></td>
<td><?php echo $users->email; ?></td>
<td><?php echo $users->phone; ?></td>
<td>
<button class="btn btn-danger user_deleted" uid="<?php echo $users->id; ?>">Delete</button>
</td>
</tr>
<?php $i++; } ?>
</tbody>
</table>
Now we will create a jquery function and then we will take the value of button's attribute in it and then pass these values inside the modal popup with the help of ID's.
<script type="text/javascript">
$(document).on('click','.user_deleted',function(){
var id = $(this).attr('uid');
$('#user_id').val(id);
$('#modal_popup').modal({backdrop: 'static', keyboard: true, show: true});
});
</script>
Now we will create confirmation modal popup to delete user.
<div class="modal modal-danger fade" id="modal_popup">
<div class="modal-dialog modal-sm">
//create form to change user status
<form action="<?php echo base_url(); ?>users/user_deleted" method="post">
<div class="modal-content">
<div class="modal-header" style="height: 150px;">
<h4 style="margin-top: 50px;text-align: center;">Are you sure, do you delete user?</h4>
//getting value in hidden field with the hep of ID's
<input type="hidden" name="id" id="user_id" value="">
</div>
<div class="modal-footer">
<button type="button" class="btn btn-danger pull-left" data-dismiss="modal">No</button>
<button type="submit" name="submit" class="btn btn-success">Yes</button>
</div>
</div>
</form>
</div>
</div>
Step 5: Create user_deleted function in user controller (Users.php)
public function user_deleted()
{
//get hidden value in variables
$id = $this->input->post('id');
$this->db->where('id',$id);
$this->db->delete('users'); //Delete user here
//Create success measage
$this->session->set_flashdata('msg',"User has been deleted successfully.");
$this->session->set_flashdata('msg_class','alert-success');
return redirect('users');
}
Step 6:- Create success flash message in user view (users_list.php).
<?php if($error = $this->session->flashdata('msg')){ ?>
<h3 class="text-success"><?php echo $error; ?></h3>
<?php } ?>
Hope this will help our developers.
Because we believe Mantra means CodingMantra
Comments ( 0 )
SEARCH POST HERE
Support Us
Subscribe My YouTube Channel
Join Our Telegram Channel & Support Eachother
CATEGORIES
INTERVIEW QUESTIONS
PROJECT SOURCE CODE
POPULAR POSTS
Elevenstech Web Tutorials
Elevenstech Web Tutorials helps you learn coding skills and enhance your skills you want.
As part of Elevenstech's Blog, Elevenstech Web Tutorials contributes to our mission of “helping people learn coding online”.
Read More
Newsletter
Subscribe to get the latest updates from Elevenstech Web Tutorials and stay up to date