Upload and Store video using PHP
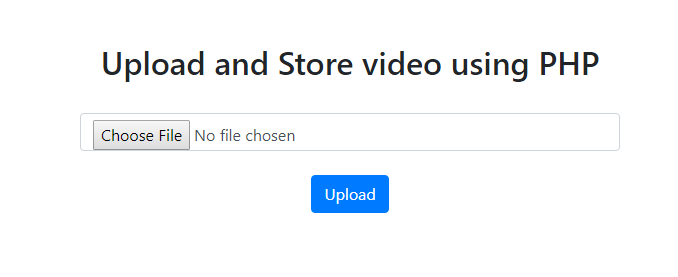
Upload and Store video using PHP
Published Apr 15,2019 by Kailash Singh
0 Comment 3131 Views
In this tutorial, we are going to learn about how to create display upload and store video using PHP.
A PHP script can be used with a HTML form to allow users to upload files to the server. Initially files are uploaded into a temporary directory and then relocated to a target destination by a PHP script.
Step 1 :- Create table in database.
CREATE TABLE `elevenstech`.`videos` ( `id` INT NOT NULL AUTO_INCREMENT , `title` VARCHAR(255) NOT NULL , `video_path` VARCHAR(255) NOT NULL , PRIMARY KEY (`id`)) ENGINE = InnoDB;
Step 2 :- Create Video Upload Page (index.php).
<form method="post" action="" enctype='multipart/form-data'>
<input type='file' name='video' class="form-control" /><br>
<input type='submit' class="btn btn-primary" value='Upload' name='upload_video'>
</form>
Step 3 :- Create connection to the database (index.php).
<?php $con = mysqli_connect('localhost','root','','elevenstech'); ?>
Step 4 :- Create Upload video folder to store videos
Step 5 :- Write Php code to upload video (index.php).
<?php
if(isset($_POST['upload_video']))
{
$maxsize = 5242880;
$title = $_FILES['video']['name'];
$image_upload1 = @end(explode('.', $title)); // get the extension
$videoFileType = strtolower($image_upload1);
$new_image_upload = time().'.'.$videoFileType; // concat the extension to another name
$image_upload_path = 'upload/'.$new_image_upload; // set folder path here to store video
$extensions_arr = array("avi","3gp","mpeg","mov","mp4");
if( in_array($videoFileType,$extensions_arr) ){
if( $_FILES['video']['size'] >= $maxsize)
{
echo "File size should be less than 5MB";
}
else{
if(move_uploaded_file($_FILES['video']['tmp_name'], $image_upload_path))
{
// insert query here
$query = "INSERT INTO videos(title,video_path) VALUES('".$title."','".$new_image_upload."')";
mysqli_query($con,$query);
echo "Upload successfully.";
}
}
}
else{
echo "You are uploading wrong file.";
}
}
?>
Step 6 :- List uploaded videos.
<?php
$sql = mysqli_query($con, "SELECT * FROM videos ORDER BY id DESC");
while($row = mysqli_fetch_assoc($sql))
{
echo "<div>";
echo "<video src='upload/".$row['video_path']."' controls width='320px' height='200px' >";
echo "</div>";
echo "<p>".$row['title']."</p><br>";
}
?>
Hope this will help our developers.
Because we believe Mantra means CodingMantra
Comments ( 0 )
Elevenstech Web Tutorials
Elevenstech Web Tutorials helps you learn coding skills and enhance your skills you want.
As part of Elevenstech's Blog, Elevenstech Web Tutorials contributes to our mission of “helping people learn coding online”.
Read More
Newsletter
Subscribe to get the latest updates from Elevenstech Web Tutorials and stay up to date